Login Form Example With Struts
This post will show you how a form process in struts 1 framework. We will create a login form and then using struts 1 we will verify the authentication of the user. This example will take following steps :
- First of all we will create a form bean (LoginForm.java) that will hold the form values provided by the user.
- Create a jsp page (Login.jsp) which will contain the form to be displayed to the user.
- Create a success page (Success.jsp) and failure page (Failure.jsp) for providing feedback to the user on their form submission.
- Create a controller helper class (LoginAction.java) that will check for the user input and decide which view to be respond to the users (Success.jsp or Failure.jsp).
- And finally we will configure our form bean and action classes in struts-config.xml.
Following is the code in LoginForm.java file:
package com.demo.form;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionMapping;
public class LoginForm extends ActionForm {
private static final long serialVersionUID = -3491637470205228033L;
private String userName = null;
private String passWord = null;
public String getUsername() {
return username;
}
public void setUsername(String userName {
this.userName = userName;
}
public String getPassword() {
return passWord;
}
public void setPassword(String passWord) {
this.passWord = passWord;
}
@Override
public void reset(ActionMapping mapping, HttpServletRequest request) {
this.passWord = null;
}
}
There are two fields in this form bean “username” and “password”, that will hold the value of two fields in the login form. One new goods here is the method “reset” which is overwritten by our form bean. “reset” method is called at the end of the every request processed by the struts. In reset method we have set the value of password as null which means every time the user will open the login jsp in browser it will show the last username but will not show the value of password.
Login.jsp
Bellow are the content of Login.jsp:
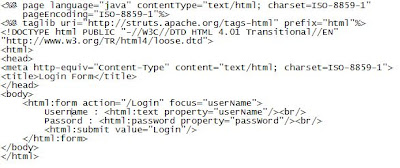
This jsp will render a form with two fields, “userName” and “passWord”. Struts HTML taglib has been used to create the form.
LoginAction.java
Lets look inside the code in LoginAction.java:
public class LoginAction extends Action {
@Override
public ActionForward execute(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
throws Exception {
LoginForm loginForm = (LoginForm)form;
if(loginForm.getUsername() == null || loginForm.getPassword() == null ||
!loginForm.getUsername().equalsIgnoreCase("abc") || !loginForm.getPassword().equals("123")){
return mapping.findForward("failure");
}
else
return mapping.findForward("success");
}
}
As you can see first of all the ActionForm instance is typecast to LoginForm in the execute method of LoginAction and then logic to verify the username and password will decide which view to be send back to the user. In this case, username must be “rahul” ans password must be “abc” to go to the view associated with the “success”, otherwise view associated with “failure” will be returned. We can see the jsp files associated with “success” and “failure”.
struts-config.xml
Entries of form beans and actions aer done in struts-config.xml as follows :
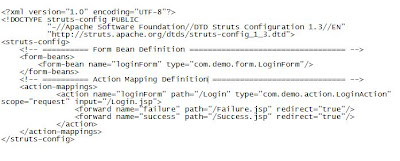
Our LoginForm class has been added as a form bean named “loginForm” and associated with the action class LoginAction. Attributes associated with the action class are specified as with the following reason :
name=”loginForm” : Struts will instantiate the LoginForm class will set the value of form properties.
path=”/Login” : The action class will be associated with the request path “/Login.do”. Hence the Login.jsp contains “/Login” as action attribute value.
input=”/Login.jsp” : Form inputs will be taken from Login.jsp.
There are two action forwards also registered with our action which tells struts that if “failure” is return by action then the response view will be “/Failure.jsp” and if “success” is returned by action then the response view will be “/Success.jsp”.
Deploy the war file in Tomcat 6 and hit the url in your browser you will get the following login form: